System administrators manage the backbone of the digital world—ensuring that systems run efficiently, securely, and without unnecessary downtime. One of the most powerful tools in a Linux system administrator’s toolkit is shell scripting. It allows repetitive tasks to be automated, saving time, reducing human error, and increasing efficiency.
This guide walks you through the basics of shell scripting and gives you 5 practical examples of tasks a Linux system administrator can automate to streamline operations.
What Is Shell Scripting?
A shell script is a text file containing a series of commands that are executed by the shell (Bash being the most popular on Linux). Think of it as a macro or automation tool that allows you to string together commands that you’d normally enter manually.
Shell scripts can perform almost any task you can perform from the command line—file operations, package installations, service monitoring, backups, and more.
Why Automate with Shell Scripting?
- Time-Saving: Run complex processes in seconds.
- Consistency: Reduce variability and human error.
- Documentation: Your scripts can serve as documentation of your standard operating procedures.
- Scalability: Easily repeat tasks across multiple systems.
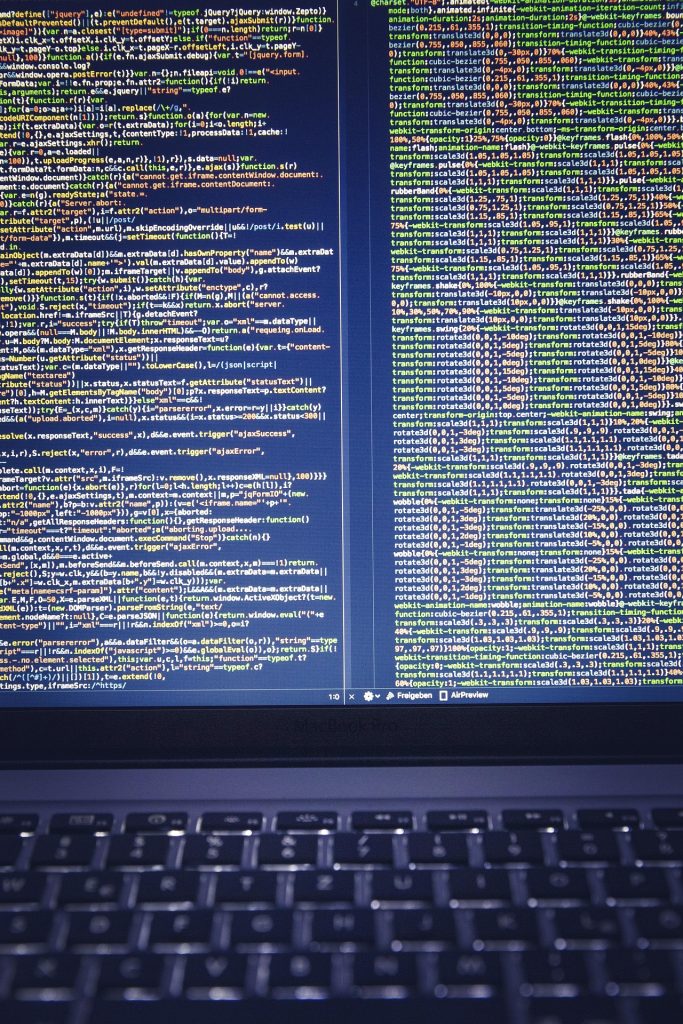
Getting Started with Shell Scripting
Before diving into examples, here’s a minimal shell script structure:
#!/bin/bash
# This is a comment
echo "Hello, world!"
#!/bin/bash
: Shebang that tells the system to use the Bash shell.echo
: Outputs text to the screen.
To make it executable:
chmod +x script.sh
# Then to run it:
./script.sh
Now, let’s dive into five useful scripts a Linux admin might use.
1. Automated System Updates
Keeping systems updated is essential for security and performance.
Script: auto_update.sh
#!/bin/bash
echo "Starting system update..."
apt-get update -y && apt-get upgrade -y
echo "System update complete!"
Why It’s Useful:
- Ensures packages are up to date.
- Can be scheduled via cron for regular maintenance.
- Saves admins from manually updating systems.
Tip: Log outputs to a file for tracking:
/auto_update.sh >> /var/log/update.log 2>&1
2. Automated Backups
Backing up important files and databases is critical. This script automates the backup of a directory to another location or remote server.
Script: backup_home.sh
#!/bin/bash
SRC="/home/user/"
DEST="/mnt/backup/home_backup_$(date +%F).tar.gz"
tar -czf $DEST $SRC
echo "Backup created at $DEST"
Why It’s Useful:
- Prevents data loss from hardware failures or accidental deletions.
- Easy to restore from a timestamped archive.
- Can be modified to use
rsync
or cloud storage (e.g., AWS S3).
3. User Account Creation
When onboarding users, creating accounts manually can be time-consuming.
Script: create_users.sh
#!/bin/bash
while read user; do
useradd -m $user
echo "$user:defaultpassword" | chpasswd
echo "User $user created."
done < user_list.txt
user_list.txt
should contain one username per line.
Why It’s Useful:
- Speeds up onboarding.
- Prevents typos in manual processes.
- Ensures uniformity in account creation.
4. Service Monitoring and Restart
Critical services like Apache, MySQL, or Docker must always be running. This script monitors and restarts them if they stop.
Script: monitor_service.sh
#!/bin/bash
SERVICE="apache2"
if systemctl is-active --quiet $SERVICE; then
echo "$SERVICE is running"
else
echo "$SERVICE is not running. Restarting..."
systemctl start $SERVICE
echo "$SERVICE restarted"
fi
Why It’s Useful:
- Ensures uptime.
- Can be run via cron or as a systemd timer.
- Sends alerts if desired (e.g., email or Slack integration).
5. Log File Rotation and Archiving
Large log files can consume disk space quickly. This script rotates and compresses logs.
Script: rotate_logs.sh
#!/bin/bash
LOG_DIR="/var/log/myapp"
ARCHIVE_DIR="/var/log/myapp/archive"
mkdir -p $ARCHIVE_DIR
for file in $LOG_DIR/*.log; do
BASENAME=$(basename $file)
mv $file $ARCHIVE_DIR/${BASENAME}_$(date +%F).log
done
gzip $ARCHIVE_DIR/*.log
Why It’s Useful:
- Prevents log files from growing indefinitely.
- Saves disk space with compression.
- Organizes logs by date.
Best Practices for Shell Scripting
- Use Comments Liberally: Document what your script is doing.
- Error Handling: Check for errors with
if
statements or exit codes. - Use Logging: Log activity for auditing and troubleshooting.
- Parameterize Your Scripts: Use variables and arguments to make scripts flexible.
- Keep It Safe: Avoid destructive commands unless you’re 100% sure. Use
echo
beforerm
.
Scheduling Scripts with Cron
To automate the execution of your scripts, use cron jobs.
Open the crontab: crontab -e
Schedule a backup every day at midnight:
0 0 * * * /home/user/scripts/backup_home.sh
Tip: Use crontab.guru
to help write cron expressions.
Debugging Your Shell Scripts
When something goes wrong:
- Run your script with
bash -x script.sh
to see each line executed. - Check file permissions.
- Use
echo
statements or logs to trace the execution.
Conclusion
Shell scripting empowers system administrators to work smarter—not harder. Whether you’re managing updates, backups, user accounts, or services, automating these tasks can free up valuable time and reduce the risk of error.
Start with the examples above, adapt them to your environment, and explore more complex scripting techniques as you grow more confident. Before long, you’ll be managing fleets of servers with ease and precision.
Power Your Projects with vpszen.com VPS Solutions
Looking for reliable hosting to run your Linux servers and host your next big project? VpsZen.com has you covered with top-tier VPS options tailored to your needs.
Choose from ARM64 VPS Servers for energy-efficient performance, or Root VPS Servers for virtual servers with dedicated resources.